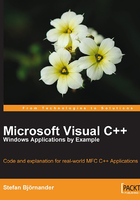
The Object-Oriented Model
The object-oriented model is very powerful. An object-oriented application consists of objects. An object exists in memory during the execution of the application. In C++, an object is defined by its class. A class can be considered a blueprint for one or more objects with the same features. A class is defined by methods and fields. A method is a function enclosed in a class. A field is a variable common to the whole class. The methods and fields of a class are together referred to as its members.
The foundation of the object-oriented theory rests on three cornerstones: part of class can be encapsulated, classes can inherit each other, and objects can be bound dynamically.
There are five relations in the object-oriented model. A class is defined in the source code, and one or more object of that class is created during the execution, the objects are instances of the class. A method of one class calls a method of the same class or another class. A class can inherit one or more other classes to reuse its functionality (a class cannot inherit itself, neither directly or indirectly). The inheriting class is called the subclass and the inherited class is called the baseclass. A class may have a member that is an object of another class; this is called aggregation. A class may also have a member pointing or referencing to an object of its own class or of another class, this is called connection.
A member of a class (field or method) may be encapsulated. There are three levels of encapsulation; public
—the member is completely accessible, protected
—the member is accessible by subclasses, and private
—the member is only accessible by the class itself. If we omit the encapsulation indicator, the member will be private. A struct
is a construction very similar to a class. The only difference is that if we omit the encapsulation indicator, the members will be public.
Similar to a local variable, a member can also be static. A static method cannot call a non-static method or access a non-static field. A static field is common to all objects of the class, as opposed to a regular field where each object has its own version of the field. Static members are not part of the object-oriented model, a static method resembles a freestanding function and a static field resembles a global variable. They are, however, directly accessible without creating any objects.
There is also single and multiple inheritance. Multiple inheritance means that a subclass can have more than one baseclass. The inheritance can also be public, protected, and private, which means that the members of the baseclass are public, protected, and private, respectively, regardless of their own encapsulation indicators. However, a member cannot become more public by a protected or public inheritance. We can say the inheritance indicator lowers (straightens) the encapsulation of the member. Private and protected inheritances are not part of the object-oriented model, and I recommend that you always use public inheritance. I also recommend that you use multiple inheritance restrictedly.
A function or a method with a pointer defined to point at an object of a class can in fact point at an object of any of its subclasses. If it calls a method that is defined in both the subclass and the baseclass and if the method of the baseclass is marked as virtual, the method of the subclass will be called. This is called dynamic binding. The methods must have the same name and parameter lists. We say the method of the baseclass is overridden by the method of the subclass. Do not confuse this with overloaded methods of the same class or of freestanding functions.
A method can also be declared as pure virtual. In that case, the class is abstract, which means that it cannot be instantiated, only inherited. The baseclass that declare's the pure virtual methods do not define them and its subclasses must either define all of its baseclasses' pure virtual methods or become abstract themselves. It is not possible to mark a class as abstract without introducing at least one pure virtual method. In fact, a class becomes abstract if it has at least one pure virtual method.