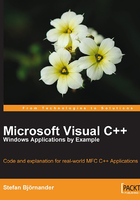
上QQ阅读APP看书,第一时间看更新
Namespaces
Code can be placed in functions and functions can be placed in classes as methods. The next step is to create a namespace
that contains classes, functions, and global variables.
namespace TestSpace { double Square(double dValue); class BankAccount { public: BankAccount(); double GetSaldo() const; void Deposit(double dAmount); void Withdraw(double dAmount); private: double m_dSaldo; }; }; double TestSpace::Square(double dValue) { return dValue * dValue; } TestSpace::BankAccount::BankAccount() :m_dSaldo(0) { // Empty. } // ... void main() { int dSquare = TestSpace::Square(3.14); TestSpace::BankAccount account; account.Deposit(1000); account.Withdraw(500); double dSaldo = account.GetSaldo(); }
We could also choose to use the namespace
. If so, we do not have to refer to the namespace
explicitly. This is what we did with the std
namespace at the beginning of Chapter 1.
#include <iostream> using namespace std; namespace TestSpace { // ... }; // ... using namespace TestSpace; void main() { cout << square(3.14); BankAccount account; account.deposit(1000); account.withdraw(500); cout << account.getSaldo(); }
Finally, namespaces can be nested. A namespace may hold another namespace, which in turn can hold another namespace and so on.
#include <iostream> using namespace std; namespace Space1 { namespace Space2 { double Square(double dValue); }; }; double Space1::Space2::Square(double dValue) { return dValue * dValue; } void main(void) { cout << Space1::Space2::Square(3); }