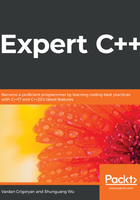
Functions as types
The second argument for unordered_map is int (*)(int, int), which literally means a pointer to a function taking two integers and returning an integer. C++ supports the class template std::function as a general-purpose function wrapper allowing us to store callable objects including ordinary functions, lambda expressions, functions objects, and so on. The stored object is referred to as the target of std::function and if it doesn't have a target, it will throw the std::bad_function_call exception if invoked. This helps us both to make the operations hash table accept any callable object as its second parameter and to handle exceptional cases such as invalid character input, mentioned earlier.
The following code block illustrates this:
#include <functional>
#include <unordered_map>
// add, subtract, multiply and divide declarations omitted for brevity
int main() {
std::unordered_map<char, std::function<int(int, int)> > operations;
operations['+'] = &add;
// ...
}
Notice the argument for std::function; it has the form int(int, int) rather than int(*)(int, int). Using std::function helps us to handle exceptional situations. For example, calling operations['x'](num1, num2); will lead to, creation of the empty std::function mapped to the character x.
And calling it will throw an exception, so we can ensure the safety of the code by properly handling the call:
// code omitted for brevity
std::cin >> num1 >> num2 >> op;
try {
operations[op](num1, num2);
} catch (std::bad_function_call e) {
// handle the exception
std::cout << "Invalid operation";
}
Finally, we can use lambda expressions— unnamed functions constructed in place and able to capture variables in scope. For example, instead of declaring the preceding functions and then inserting them into the hash table, we can create a lambda expression right before inserting it into the hash table:
std::unordered_map<char, std::function<int(int, int)> > operations;
operations['+'] = [](int a, int b) { return a + b; }
operations['-'] = [](int a, int b) { return a * b; }
// ...
std::cin >> num1 >> num2 >> op;
try {
operations[op](num1, num2);
} catch (std::bad_functional_call e) {
// ...
}
Lambda expressions will be covered throughout the book.