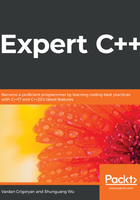
Working with classes
Classes make things a lot easier when dealing with objects. They do the simplest necessary thing in OOP: they combine data with functions for manipulating data. Let's rewrite the example of the Product struct using a class and its powerful features:
class Product {
public:
Product() = default; // default constructor
Product(const Product&); // copy constructor
Product(Product&&); // move constructor
Product& operator=(const Product&) = default;
Product& operator=(Product&&) = default;
// destructor is not declared, should be generated by the compiler
public:
void set_name(const std::string&);
std::string name() const;
void set_availability(bool);
bool available() const;
// code omitted for brevity
private:
std::string name_;
double price_;
int rating_;
bool available_;
};
std::ostream& operator<<(std::ostream&, const Product&);
std::istream& operator>>(std::istream&, Product&);
The class declaration seems more organized, even though it exposes more functions than we use to define a similar struct. Here's how we should illustrate the class:
The preceding image is somewhat special. As you can see, it has organized sections, signs before the names of functions, and so on. This type of diagram is called a Unified Modeling Language (UML) class diagram. UML is a way to standardize the process of illustrating classes and their relationship. The first section is the name of the class (in bold), next comes the section for member variables, and then the section for member functions. The + (plus) sign in front of a function name means that the function is public. Member variables are usually private, but, if you need to emphasize this, you can use the - (minus) sign. We can omit all the details by simply illustrating the class, as shown in the following UML diagram:
We will use UML diagrams throughout this book and will introduce new types of diagrams as needed. Before dealing with initializing, copying, moving, default and deleted functions, and, of course, operator overloading, let's clear a couple of things up.