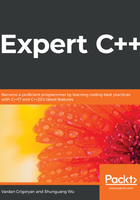
Lvalue references
Before understanding why rvalue references were introduced in the first place, let's clear things up regarding lvalues, references, and lvalue-references. When a variable is an lvalue, it can be addressed, it can be pointed to, and it has a scoped storage duration:
double pi{3.14}; // lvalue
int x{42}; // lvalue
int y{x}; // lvalue
int& ref{x}; // lvalue-reference
ref is an lvalue reference, a synonym for a variable that can be treated as a const pointer:
int * const ref = &x;
Besides the ability to modify the objects by a reference, we pass heavy objects to functions by reference in order to optimize and avoid redundant object copies. For example, the operator+ for the Warehouse takes two objects by reference, thus making it copy addresses of objects rather than full objects.
Lvalue references optimize the code in terms of function calls, but, to optimize temporaries, we should move on to rvalue references.