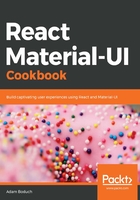
There's more...
When you have a lot of drawer items and sections, you can still overwhelm your users with the amount of information to parse. One solution is to have collapsible sections. For this, you can add a Button component to the ListSubheader component so that it's clickable.
Here's what the code looks like:
<ListSubheader>
<Button
disableRipple
classes={{ root: classes.listSubheader }}
onClick={toggleSection('cpu')}
>
CPU
</Button>
</ListSubheader>
The ripple effect that would normally happen when you click on a button is disabled here because you want the header text to still look like header text. This also requires a little bit of CSS customization in the listSubheader class:
const styles = theme => ({
alignContent: {
alignSelf: 'center'
},
listSubheader: {
padding: 0,
minWidth: 0,
color: 'inherit',
'&:hover': {
background: 'inherit'
}
}
});
When the section header button is clicked, it toggles the state of the section, which in turn, toggles the visibility of the section items. Here's the toggleSection() function:
const toggleSection = name => () => {
setSections({ ...sections, [name]: !sections[name] });
};
This is a higher-order function that returns a new function as the onClick handler for the button. The name argument is the name of the section state to toggle.
Here's the new state that was added to support toggling sections:
const [sections, setSections] = useState({
cpu: true,
memory: false,
storage: false,
network: false
});
When the screen first loads, the CPU section will be the only section with visible items since it's the only state that's true. Next, let's look at how the ListItems are actually collapsed when their corresponding section state is false:
const ListItems = ({ items, visible, onClick }) => (
<Collapse in={visible}>
{items
.filter(({ hidden }) => !hidden)
.map(({ label, disabled, Icon }, i) => (
<ListItem
button
key={i}
disabled={disabled}
onClick={onClick(label)}
>
<ListItemIcon>
<Icon />
</ListItemIcon>
<ListItemText>{label}</ListItemText>
</ListItem>
))}
</Collapse>
);
The ListItems component now accepts a visible property. This is used by the Collapse component, which will hide its children using a collapsing animation when hiding components. Finally, here's how the new ListItems component is used:
<ListItems
visible={sections.cpu}
items={items.cpu}
onClick={onClick}
/>
When the screen first loads, and you click on the SHOW DRAWER button, you should see something similar to this:

There's way less information for the user to parse now. They can click on the section headers to see the list items, and they can click again to collapse the section; for example, they could collapse the CPU section and expand the MEMORY section:
