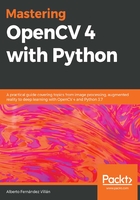
Saving camera frames
This previous example can be easily modified to add a useful functionality. Imagine that you want to save some frames to disk when something interesting happens. In the following example, read_camera_capture.py, we are going to add this functionality. When the C key is pressed on the keyboard, we save the current frame to disk. We save both the BGR and the grayscale frames. The code that performs this functionality is shown here:
# Press c on keyboard to save current frame
if cv2.waitKey(20) & 0xFF == ord('c'):
frame_name = "camera_frame_{}.png".format(frame_index)
gray_frame_name = "grayscale_camera_frame_{}.png".format(frame_index)
cv2.imwrite(frame_name, frame)
cv2.imwrite(gray_frame_name, gray_frame)
frame_index += 1
ord('c') returns the value representing the c character using eight bits. Additionally, the cv2.waitKey() value is bitwise AND using the & operator with 0xFF to get only its last eight bits. Therefore, we can perform a comparison between these two 8-bit values. When the C key is pressed, we build the names for both frames. Then, we save the two images to disk. Finally, frame_index is incremented so that it's ready for the next frame to be saved. Check out read_camera_capture.py to see the full code of this script.