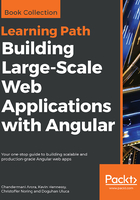
Angular directives
As a framework, Angular tries to enhance the HTML DSL (short for Domain-Specific Language):
- Components are referenced in HTML using custom tags such as <abe-workout-runner></abe-workout-runner> (not part of standard HTML constructs). This highlights the first extension point.
- The use of [] and () for property and event binding defines the second.
- And then there are directives, the third extension point which are further classified into attribute and structural directives, and components (components are directive too!).
While components come with their own view, attribute directives are there to enhance the appearance and/or behavior of existing elements/components.
Structural directives do not have their own view too; they change the DOM layout of the elements on which they are applied. We will dedicate a complete section later in the chapter to understanding these structural directives.
The ngStyle directive used in the workout-runner view is, in fact, an attribute directive:
<div class="progress-bar" role="progressbar" [ngStyle] = "{'width':(exerciseRunningDuration/currentExercise.duration) * 100 + '%'}"></div>
The ngStyle directive does not have its own view; instead, it allows us to set multiple styles (width in this case) on an HTML element using binding expressions. We will be covering a number of framework attribute directives later in this book.
Directives is an umbrella term used for component directives (also known as components), attribute directives, and structural directives. Throughout the book, when we use the term directive, we will be referring to either an attribute directive or a structural directive depending on the context. Component directives are always referred to as components.
With a basic understanding of the directive types that Angular has, we can comprehend the process of target selection for binding.