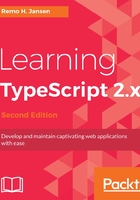
Type inference
TypeScript tries to find the types of the variables and objects in our application using what is known as type inference. When we declare a variable, TypeScript will try to observe the value assigned to the variables in the application to identify its type. Let's examine some examples:
let myVariable1 = 3;
The type of the variable myVariable1 is inferred as a number.
let myVariable2 = "Hello";
The type of the variable myVariable2 is inferred as a string.
let myVariable3 = {
name: "Remo",
surname: "Jansen",
age: 29
};
The type of the variable myVariable3 is inferred as the following type:
{ name: string; surname: string; age: number; }
The type any is assigned in the cases in which TypeScript is not able to identify the type of a variable. For example, given the following function:
function add(a, b) {
return a + b;
}
The type of the function add is inferred as the following type:
(a: any, b: any) => any;
The type any is problematic because it prevents the TypeScript compiler from identifying many potential errors. Fortunately, TypeScript features optional type annotations that can be used to solve this problem.