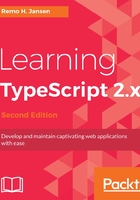
Non-nullable types
TypeScript 2.0 introduced what are known as non-nullable types. TypeScript used to consider null and undefined to be valid values of every type.
The following diagram represents the values that can be assigned to the type number when non-nullable types are disabled:

As we can see in the preceding diagram, undefined and null are allowed as values of the type number, together with the NaN value and all the possible numbers.
The following code snippet demonstrates how undefined and null are allowed as values of the type number when non-nullable types are disabled:
let name: string;
name = "Remo"; // OK
name = null; // OK
name = undefined; // OK
The same can be said about all other types:
let age: number;
age = 28; // OK
age = null; // OK
age = undefined; // OK
let person: { name: string, age: number};
person = { name: "Remo", age: 28 }; // OK
person = { name: null, age: null }; // OK
person = { name: undefined, age: undefined }; // OK
person = null; // OK
person = undefined; // OK
When non-nullable types are enabled, the values null and undefined are considered independent types and stop being considered as valid values of the type number:

The following code snippet demonstrates how undefined and null are not allowed as values of the type number when non-nullable types are enabled:
let name: string;
name = "Remo"; // OK
name = null; // Error
name = undefined; // Error
The same can be said about all other types:
let age: number;
age = 28; // OK
age = null; // Error
age = undefined; // Error
let person: { name: string, age: number};
person = { name: "Remo", age: 28 }; // OK
person = { name: null, age: null }; // Error
person = { name: undefined, age: undefined }; // Error
person = null; // Error
person = undefined; // Error
We can enable non-nullable types by using the --strictNullChecks compilation flag:
tsc -strictNullChecks file.ts
When non-nullable types are enabled, we can use union types to create nullable versions of a type:
type NullableNumber = number | null;