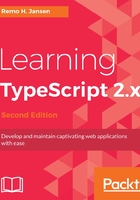
Functions with default parameters
When a function has some optional parameters, we must check whether an argument has been passed to the function (just like we did in the previous example) to prevent potential errors.
There are some scenarios where it would be more useful to provide a default value for a parameter when it is not supplied than making it an optional parameter. Let's rewrite the add function (from the previous section) using the inline if structure:
function add(foo: number, bar: number, foobar?: number): number { return foo + bar + (foobar !== undefined ? foobar : 0); }
There is nothing wrong with the preceding function, but we can improve its readability by providing a default value for the foobar parameter instead of using an optional parameter:
function add(foo: number, bar: number, foobar: number = 0): number { return foo + bar + foobar; }
To indicate that a function parameter is optional, we need to provide a default value using the = operator when declaring the function's signature. After compiling the preceding code examples, the TypeScript compiler will generate an if structure in the JavaScript output to set a default value for the foobar parameter if it is not passed as an argument to the function:
function add(foo, bar, foobar) { if (foobar === void 0) { foobar = 0; } return foo + bar + foobar; }
This is great because the TypeScript compiler generated the code necessary for us to prevent potential runtime errors.
function test() {
var undefined = 2; // 2
console.log(undefined === 2); // true
}
Just like optional parameters, default parameters must always be located after any required parameters in the function's parameter list.