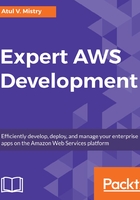
Benefits and features of Amazon SQS
Let's explore a few benefits of Amazon SQS:
- Operational efficiency: Amazon SQS will help to eliminate the administrative overhead and complexity associated with infrastructure and dedicated message-oriented middleware (MoM).
There isn't any upfront cost. No need to install, acquire, or configure any messaging software.
With Amazon SQS, message queues are created dynamically and scale automatically to build applications quickly.
- Reliability: Amazon SQS helps to transmit any amount of data, at any throughput, without losing messages. To increase the overall fault tolerance of the system, SQS can help to decouple application components to run and fail independently. SQS queues can store messages of any components in distributed applications.
Messages are stored in multiple availability zones so that they will be available whenever the application needs them.
Messages will be delivered once with the FIFO queue and at least once with the standard queue.
- Security: In Amazon SQS, applications use server-side encryption (SSE) to encrypt the message body while exchanging sensitive data. AWS Key Management Service (KMS) with Amazon SQS SSE integration allows you to manage the keys centrally. Authentication mechanisms help to secure the messages stored in SQS message queues.
- Integration: AWS services can easily integrate with Amazon SQS to build scalable and flexible applications. You can integrate Amazon EC2, Amazon Simple Storage Service (Amazon S3), Amazon DynamoDB, Amazon RDS, Amazon EC2 Container Service (Amazon ECS), and AWS Lambda.
Amazon SQS works very well with Amazon Simple Notification Service (SNS) for a powerful messaging solution.
- Productivity: You can start Amazon SQS message queuing using a console or SDK. SQS has four APIs which you can easily add: CreateQueue, SendMessage, ReceiveMessage, and DeleteMessage. You can use the same APIs in standard queues or FIFO queues.
- Scalability: It scales elastically and manages pre-provisioning and capacity planning with the application. Costs to use Amazon SQS are based on use and not on an always-on model.
Amazon SQS provides the following major features:
- Redundant infrastructure: It provides high availability to produce and consume messages and highly-concurrent to access the messages.
- Multiple producers and consumers: Multiple consumers or producers are available in your system at the same time.
- Configurable settings per queue: All of your queues may have different configuration settings for example, if one queue requires more time for processing than others.
- Variable message size: Message size is 256 KB. Large messages can be split into smaller ones. Large message content can be stored in Amazon S3 or Amazon DynamoDB.
- Access control: Sender and receiver can be controlled.
- Delay queues: Default delay can be set on a queue so that it will delay enqueued messages for a specified time.
To use the AWS SDK for Amazon SQS, it has the same setup and configuration which we have discussed in Chapter 1, AWS Tools and SDKs. Here I will use Eclipse IDE for the example.
Let's explore an example of Amazon SQS:
- Open Eclipse IDE and create a new AWS Java project. Add information such as Project name, Group ID, Artifact ID, Version, and Package Name.
- Crate a new Java class under this project and import a few classes to use the Amazon SQS.
- AmazonSQS and AmazonSQSClientBuilder are used to create an instance of sqsclient. Other imports are used to create and delete queues, and to send, receive, and delete messages:
AmazonSQS sqsClient = AmazonSQSClientBuilder.standard()
.withCredentials(credentials)
.withRegion(Regions.US_WEST_2).build();
An sqsClient object will be created from AmazonSQSClientBuilder. It needs to set the credentials and region where you want to create the SQS.
- MyDemoQueue will be created from the CreateQueueRequest class. You will get the queue URL from the sqsClient by calling the getQueueUrl method of createQueue:
CreateQueueRequest createQueue = new CreateQueueRequest("MyDemoQueue");
String myDemoQueueUrl = sqsClient.createQueue(createQueue).getQueueUrl();
- New messages will be sent from sqsClientby calling the SendMessageRequest class:
sqsClient.sendMessage(new SendMessageRequest(myDemoQueueUrl, "This is SQS Demo Example Test Message."));
- receiveMessageRequestTest will receive messages from MyDemoQueue:
ReceiveMessageRequest receiveMessageRequest = new ReceiveMessageRequest(myDemoQueueUrl);
- It will create the instance of messageList and get information such as message ID, body, and so on:
List<Message> messageList = sqsClient.receiveMessage(receiveMessageRequest).getMessages();
for (Message msgInfo : messageList) {
System.out.println("MessageId:" + msgInfo.getMessageId());
System.out.println("ReceiptHandle:"+ msgInfo.getReceiptHandle());
System.out.println("MD5 Of Body:" + msgInfo.getMD5OfBody());
System.out.println("Body: " + msgInfo.getBody());
}
- You can delete the message by calling the deleteMessage method of sqsClient:
String messageReceiptHandle = messageList.get(0).getReceiptHandle();
sqsClient.deleteMessage(new DeleteMessageRequest(myDemoQueueUrl, messageReceiptHandle));
- You can delete the queue by calling the deleteQueue method of sqsClient:
sqsClient.deleteQueue(new DeleteQueueRequest(myDemoQueueUrl));