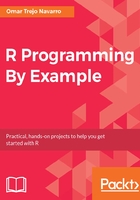
Logicals
Logical vectors contain Boolean values, which can only be TRUE or FALSE. When you want to create logical variables with such values, you must avoid using quotation marks around them and remember that they are all capital letters, as shown here. When programming in R, logical values are commonly used to test a condition, which is in turn used to decide which branch from a complex program we should take. We will look at examples for this type of behavior in a later section in this chapter:
x <- TRUE
In R, you can easily convert values among different types with the as.*() functions, where * is used as a wildcard which can be replaced with character, numeric, or logical to convert among these types. The functions work by receiving an object of a different type from what the function name specifies and return the object parsed into the specified type if possible, or return an NA if it's not possible. The following example shows how to convert the TRUE string into a logical value, which in this case non-surprisingly turns out to be the logical TRUE:
as.logical("TRUE")
#> [1] TRUE
Converting from characters and numerics into logicals is one of those things that is not very intuitive in R. The following table shows some of this behavior. Note that even though the true string (all lowercase letters) is not a valid logical value when removing quotation marks, it is converted into a TRUE value when applying the as.logical() to it, for compatibility reasons. Also note that since T is a valid logical value, which is a shortcut for TRUE, it's corresponding text is also accepted as meaning such a value. The same logic applies to false and F. Any other string will return an NA value, meaning that the string could not be parsed as a logical value. Also note that 0 will be parsed as FALSE, but any other numeric value, including Inf, will be converted to a TRUE value. Finally, note that both NA and NaN will be parsed, returning NA in both cases.
The as.character() and as.numeric() functions have less counter-intuitive behavior, and I will leave you to explore them on your own. When you do, try to test as many edge cases as you can. Doing so will help you foresee possible issues as you develop your own programs.
Before we move on, you should know that these data structures can be organized by their dimensionality and whether they're homogeneous (all contents must be of the same type) or heterogeneous (the contents can be of different types). Vectors, matrices, and arrays are homogeneous data structures, while lists and data frames are heterogeneous. Vectors and lists have a single dimension, matrices and data frames have two dimensions, and arrays can have as many dimensions as we want.
When it comes to dimensions, arrays in R are different from arrays in many other languages, where you would have to create an array of arrays to produce a two-dimensional structure, which is not necessary in R.