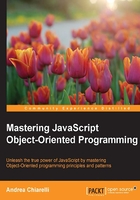
Abstraction and modeling support
The first requirement for us to consider a language as Object Oriented is its support to model a problem through objects. We already know that JavaScript supports objects, but here we should determine whether they are supported in order to be able to model reality.
In fact, in Object-Oriented Programming we try to model real-world entities and processes and represent them in our software. We need a model because it is a simplification of reality, it allows us to reduce the complexity offering a vision from a particular perspective and helps us to reason about a relationship among entities.
This simplification feature is usually known as abstraction, and it is sometimes considered one of the principles of OOP. Abstraction is the concept of moving the focus from the details and concrete implementation of things to the features that are relevant for a specific purpose, with a more general and abstract approach. In other words, abstraction is the capability to define which properties and actions of a real-world entity have to be represented by means of objects in a program in order to solve a specific problem.
For example, thanks to abstraction, we can decide that to solve a specific problem we can represent a person just as an object with name, surname, and age, since other information such as address, height, hair color, and so on are not relevant for our purpose.
More than a language feature, it seems a human capability. For this reason, we prefer not to consider it an OOP principle but a (human) capability to support modeling.
Modeling reality not only involves defining objects with relevant features for a specific purpose. It also includes the definition of relationships between objects, such as Association, Aggregation, and Composition.
Association
Association is a relationship between two or more objects where each object is independent of each other. This means that an object can exist without the other and no object owns the other.
Let us clarify with an example. In order to define a parent-child relationship between persons, we can do so as follows:
function Person(name, surname) {
this.name = name;
this.surname = surname;
this.parent = null;
}
var johnSmith = new Person("John", "Smith");
var fredSmith = new Person("Fred", "Smith");
fredSmith.parent = johnSmith;
The assignment of the object johnSmith
to the parent
property of the object fredSmith
establishes an association between the two objects. Of course, the object johnSmith
lives independently from the object fredSmith
and vice versa. Both can be created and deleted independently to each other.
As we can see from the example, JavaScript allows us to define association between objects using a simple object reference through a property.
Aggregation
Aggregation is a special form of association relationship where an object has a major role than the other one. Usually, this major role determines a sort of ownership of an object in relation to the other. The owner object is often called aggregate and the owned object is called component. However, each object has an independent life.
An example of an aggregation relationship is the one between a company and its employees, as in the following example:
var company = { name: "ACME Inc.", employees: [] }; var johnSmith = new Person("John", "Smith"); var marioRossi = new Person("Mario", "Rossi"); company.employees.push(johnSmith); company.employees.push(marioRossi);
The person objects added to the employees
collection help define the company object, but they are independent from it. If the company object is deleted, each single person still lives. However, the real meaning of a company is bound to the presence of its employees.
Again, the code show us that the aggregation relationship is supported by JavaScript by means of object reference.
It is important not to confuse the Association with the Aggregation. Even if the support of the two relationships is syntactically identical, that is, the assignment or attachment of an object to a property, from a conceptual point of view they represent different situations.
Aggregation is the mechanism that allows you to create an object consisting of several objects, while the association relates autonomous objects.
In any case, JavaScript makes no control over the way in which we associate or aggregate objects between them. Association and Aggregation raise a constraint more conceptual than technical.
Composition
Composition is a strong type of Aggregation, where each component object has no independent life without its owner, the aggregate. Consider the following example:
var person = {name: "John", surname: "Smith", address: { street: "123 Duncannon Street", city: "London", country: "United Kingdom" }};
This code defines a person with his address represented as an object. The address
property is strictly bound to the person
object. Its life is dependent on the life of the person and it cannot have an independent life without the person. If the person
object is deleted, also the address
object is deleted.
In this case, the strict relation between the person and their address is expressed in JavaScript assigning directly the literal representing the address to the address
property.