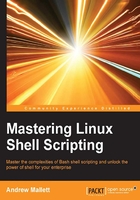
Enhancing learning with simple scripts
Our scripts are still a little trivial and we have not looked at conditional statements, so we can test for correct input, but let's take a look at some simple scripts that we can build with some functionality.
Backing-up with scripts
Now that we have created some scripts, we may want to back these up in a different location. If we create a script to prompt us, we can choose the location and the type of files that we want to backup.
Consider the following script for your first practice. Create the script and name it as $HOME/backup.sh
:
#!/bin/bash # Author: @theurbanpenguin # Web: www.theurbapenguin.com # Script to prompt to back up files and location # The files will be search on from the user's home # directory and can only be backed up to a directory # within $HOME # Last Edited: July 4 2015 read -p "Which file types do you want to backup " file_suffix read -p "Which directory do you want to backup to " dir_name # The next lines creates the directory if it does not exist test -d $HOME/$dir_name || mkdir -m 700 $HOME/$dir_name # The find command will copy files the match the # search criteria ie .sh . The -path, -prune and -o # options are to exclude the backdirectory from the # backup. find $HOME -path $HOME/$dir_name -prune -o \ -name "*$file_suffix" -exec cp {} $HOME/$dir_name/ \; exit 0
You will see that the file is commented; though as black and white the readability is a little difficult. If you have an electronic copy of the book, you should see the colors in the following screenshot:

As the script runs, you may choose .sh
for the files to backup and backup
as the directory. The script execution is shown in the following screenshot along with a listing of the directory:

Now you can see if we can start to create meaningful scripts with trivial scripting; although I will strongly urge adding error checking the user input if this script has to be for something other than personal use. As we progress into the book will cover this.
Connecting to a server
Let's look at some practical scripts that we can use to connect to servers. First, we will look at ping and in the second script we will look at prompting for SSH credentials.
Version 1 – ping
This is something we all can do as no special services are required. This will simplify the ping
command for console users who may not know the details of the command. This will ping the server for just three counts rather than the normal infinite amount. There is no output if the server is alive but a failed server reports Sever dead
. Create the script as $HOME/bin/ping_server.sh
:
#!/bin/bash # Author: @theurbanpenguin # Web: www.theurbapenguin.com # Script to ping a server # Last Edited: July 4 2015 read -p "Which server should be pinged " server_addr ping -c3 $server_addr 2>&1 > /dev/null || echo "Server dead"
The following screenshot shows successful and failed output:

Version 2 – SSH
Often SSH is installed and running on servers, so you may be able to run this script if your system is running SSH or you have access to an SSH server. In this script, we prompt for the server address and username and pass them through to the SSH client. Create the script as $HOME/bin/connect_server.sh
:
#!/bin/bash # Author: @theurbanpenguin # Web: www.theurbapenguin.com # Script to prompt fossh connection # Last Edited: July 4 2015 read -p "Which server do you want to connect to: " server_name read -p "Which username do you want to use: " user_name ssh ${user_name}@$server_name
Tip
Note the use of the brace bracket to delimit the variable from the @
symbol in the last line of the script.
Version 3 – MySQL/MariaDB
In the next script, we will provide the detail for a database connection along with the SQL query to execute. You will be able to run this if you have a MariaDB or MySQL database server on your system or one that you can connect to. For the demonstration, I will use a Raspberry Pi running Ubuntu-Mate 15.04 and MariaDB version 10; however, this should work for any MySQL server or MariaDB from version 5 onwards. The script collects user and password information as well as the SQL command to execute. Create the script as $HOME/bin/run_mql.sh
:
#!/bin/bash # Author: @theurbanpenguin # Web: www.theurbapenguin.com # Script to prompt for MYSQL user password and command # Last Edited: July 4 2015 read -p "MySQL User: " user_name read -sp "MySQL Password: " mysql_pwd echo read -p "MySQL Command: " mysql_cmd read -p "MySQL Database: " mysql_db mysql -u $user_name -p$mysql_pwd$mysql_db -e"$mysql_cmd"
In the script, we can see that we suppress the display of the MySQL password when we input it into the read
command using the -s
option. Again, we use echo
directly to ensure that the next prompt starts on a new line.
The script input is shown in the following screenshot:

Now, we can easily see the password suppression working and the ease of adding to the MySQL commands.